As we have already discussed about launching the Google page from Selenium WebDriver in Selenium Part 1 Blog, let us understand the Selenium concepts of ‘Navigation’ and ‘Locators’ in this article.
Navigation:
To understand the launching examples let me use the same script of part 1.
import unittest
from selenium import webdriver
from selenium.webdriver.common.desired_capabilities import DesiredCapabilities
driver = webdriver.Chrome("C:\\Users\\amurthy\\PycharmProjects\\firstSeleniumTest\\ve
nv\\Driver\\chromedriver.exe")
driver.get("https://google.com")
driver.find_element_by_name("q").send_keys("Automation step by step")
As already explained, driver.get(“https://google.com”) launches the URL.
Webdriver will wait until the page has fully loaded, once loading event is filed. Next is to identify the HTML elements within the page.
How to Navigate forward or backward in your browser’s history?
driver.forward() driver.back()
Cookies:
Let us understand, how to set and get cookies.
driver.get("http://www.google.com")
# Now set the cookie. This one's valid for the entire domain
cookie = {‘name’ : ‘foo’, ‘value’ : ‘bar’}
driver.add_cookie(cookie)
# And now output all the available cookies for the current URL
driver.get_cookies()
Locators:
‘Locators’ is the command that tells Selenium WebDriver to identify elements.
It provides the following methods to locate elements on the page.
1. find_element_by_id
2. find_element_by_name
3. find_element_by_XPath
4. find_element_by_link_text
5. find_element_by_partial_link_text
6. find_element_by_tag_name
7. find_element_by_class_name
8. find_element_by_css_selector
Locating by ID:
Consider the below script as an example
< input type="checkbox" name="remember" id="remember" class="form-check-input" >,
Here we can see the tag of ID and as IDs are unique for each element;
Element can be located as
driver.find_element_by_id(“remember”)
Locating by Name:
HTML name attribute may or may not be unique for elements. When we try locating elements by name, the first element whose name attribute matches the name being located is returned.
<input name="continue" type="submit" value="Login" />
The element can be located as
driver.find_element_by_name(“continue”)
Locating by XPath:
XPath is the language used for locating nodes. It uses path expression to select nodes or node sets in an XML document.
The advantage of using XPath is that it can access almost all the elements, even those without class, name or id attributes.
Let us understand XPath terminology:
Nodes: It has seven types of nodes
- Element
- Attribute
- Text
- Namespace
- Processing-instruction
- Comment
- Document
The Nodes are selected by following a path or steps.
- /[Absolute XPath] > select from root nodes
- //[Relative XPath] > select nodes in document from the current node that match the selection no matter where they are
- . > selects the current nodes
- .. > selects the parent of the current node
- @ > selects attributes
Difference between Absolute XPath and Relative XPath
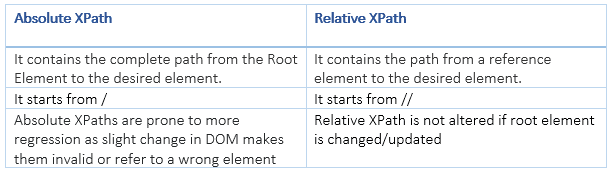
Now, let us take a look at how we can locate elements using XPath in Selenium.
Consider the below HTML snippet,
<body>
<form id="loginForm">
<input name="username" type="text" />
<input name="password" type="password" />
<input name="continue" type="submit" value="Login" />
<input name="continue" type="button" value="Clear" />
</form>
</body>
<html>
The form elements can be located as:
login_form = driver.find_element_by_XPath("/html/body/form[1]")
login_form = driver.find_element_by_XPath("//form[1]")
login_form = driver.find_element_by_XPath("//form[@id='loginForm']")
login_form = driver.find_element_by_XPath("/html/body/form[1]")
login_form = driver.find_element_by_XPath("//form[1]")
login_form = driver.find_element_by_XPath("//form[@id='loginForm']")
The Username element can be located as:
username = driver.find_element_by_XPath("//form[input/@name='username']")
username = driver.find_element_by_XPath("//form[@id='loginForm']/input[1]")
username = driver.find_element_by_XPath("//input[@name='username']")
- First form element with an input child element with attribute named name and the value username
- First input child element of the form element with attribute named id and the value loginForm
- First input element with attribute named ‘name’ and the value username
The “Clear” button element can be located as :
clear_button = driver.find_element_by_XPath("//input[@name='continue' [@type='button']")
clear_button = driver.find_element_by_XPath("//form[@id='loginForm']/input[4]")
Locating Hyperlink by Link Text:
This type of locator applies only to hyperlink text, when we use link text used with anchor tag. The first element with link text value matching location will be returned.
Consider below HTML snippet for reference,
<html>
<body>
<p>Are you sure you want to do this?</p>
<a href="continue.html">Continue</a>
<a href="cancel.html">Cancel</a>
</body>
<html>
The continue .html link can be located like this:
continue_link = driver.find_element_by_link_text('Continue')
continue_link = driver.find_element_by_partial_link_text('Conti')
Locating Elements by Tag Name
Elements can be located by Tag name. The first element with given tag name will be returned.
Consider below HTML snippet for reference.
<html>
<body>
<h1>Welcome</h1>
<p>Site content goes here.</p>
</body>
<html>
Element can be located by
heading1 = driver.find_element_by_tag_name('h1')
Locating Elements by Class Name:
Elements can be located by class attribute name. The first element with the matching class attribute name will be returned.
Consider below HTML snippet for reference,
<html>
<body>
<p class="content">Site content goes here.</p>
</body>
<html>
The “p” element can be located like this:
content = driver.find_element_by_class_name('content')
Locating Elements by CSS Selectors:
A CSS Selector is a combination of an element selector and a value which identifies the web element within a web page. They are string representations of HTML tags, attributes, IDs and Classes.
Let us understand, by comparing with XPath.
Direct child
A direct child in XPath is defined by the use of a “/“, in CSS, it is defined using “>”
XPath: //div/a
CSS: div > a
Child or Sub-Child
If an element could be within another element or one of its child’s, it is defined in XPath using “//” and in CSS just by a whitespace.
XPath: //div//a
CSS: div a
1. ID: An element’s id in Xpath is defined using: “[@id=’example’]” and in CSS using: “#” – IDs must be unique within the DOM.
XPath: //div[@id='example']
CSS: #example
2. Class: An element’s class in Xpath: “[@class=’example’]” while in CSS it’s just “.” XPath: //div[@class='example']
CSS: .example
Let us understand, how to write CSS-selector in Selenium
Consider following HTML snippet for reference,
<html>
<body>
<p class="content">Site content goes here.</p>
</body>
<html>
The ‘p’ element can be located like this
content = driver.find_element_by_css_selector('p.content')
Conclusion
This article is about the different ways to locate elements within a web page. With Selenium becoming a widely popular option for developers to run automation tests for web applications, hope this article helps you learn a few things. Upcoming articles will be on the usage of these locators to perform GUI testing. Stay tuned!