Modern JavaScript is really a wide and moving target. You may have learned JavaScript five years ago and some of those techniques may be considered old by today’s standards. But then, some other techniques older than these may still be considered as the modern best practices. So, here the intent is to guide you through various modern techniques of JavaScript and features of ES6.
What is ES6?
ECMAScript 2015, also known as ES6, is a fundamental version of the ECMAScript standard. JavaScript ES6 brings new syntax and awesome features to make your code more modern and readable. It allows you to write less code and do more. ES6 introduces us to many great features like arrow functions, template strings, class destruction, modules and many more.
New ES6 Features that will be covered in this article are:
- Arrow Functions: Lexical ‘this’ Keyword
- Promises
- Generators
- Default Parameters
- Rest parameter and Spread operator
- Template Literals
- For-of loop
- New String methods
- New Object methods
1. Arrow Functions
An arrow function expression is a syntactically compact alternative to a regular function expression, although without its bindings to this, arguments, super, or new. target keywords.
Example:
const something = () => doSomething()
2. Promises
Promises allow us to eliminate the famous “callback hell”, although they introduce a bit more complexity (which has been solved in ES2017 with async, a higher-level construct).
Example:
const wait = () => new Promise((resolve, reject) => {
resolve(‘Learn Javascript Deeply!’);
})
wait().then((data) => {
console.log(data); // Learn Javascript Deeply
})
3. Generators
Generator is a special kind of function with the ability to pause itself, and resume later, allowing other code to run in the meantime. The function* declaration (function keyword followed by an asterisk) defines a generator function, which returns a Generator object.
Example:
function* generator(i) {
yield i;
yield i + 10;
}
const gen = generator(10);
console.log(gen.next().value); // expected output: 10
console.log(gen.next().value); // expected output: 20
4. Default Parameters
Functions now support default parameters.
Example:
const someFunction = function(index = 0, testing = true) { /* ... */ }
someFunction()
5. Rest Parameter and Spread Operator
The rest parameters are used to get the argument of an array, and return a new array.
Example:
function sum(...theArgs) {
return theArgs.reduce((previous, current) => {
return previous + current;
});
}
console.log(sum(1, 2, 3));
You can expand an array, an object or a string using the spread operator …
Example:
const a = [1, 2, 3]
You can create a new array using
const b = [...a, 4, 5, 6]
6. Template Literals
They provide a way to embed expressions into strings, effectively inserting the values, by using the ${a_variable} syntax.
Example:
const joe = 'test'
const string = `something ${joe}` //something test
7. For-off loop
ES5, back in 2009, introduced forEach() loops. Although it was good, it offered no way to break, like for loops always did. ES2015 introduced the for-of loop, which combines the conciseness of forEach with the ability to break.
Example:
//get the index as well, using `entries()`
for (const [i, v] of ['a', 'b', 'c'].entries()) {
console.log(i, v);
}
8. New String methods
String variables now have some new instance methods:
- repeat() – repeats the strings for the specified number of times: ‘Ho’.repeat(3) //HoHoHo
- codePointAt() – handles retrieving the Unicode code of characters that cannot be represented by a single 16-bit UTF-16 unit, but need 2 instead
9. New Object methods
ES6 introduced several static methods under the Object namespace:
- Object.is() – determines if two values are the same
- Object.assign() – used to shallow copy an object (a copy of the reference pointer to the object)
- Object.setPrototypeOf sets an object prototype
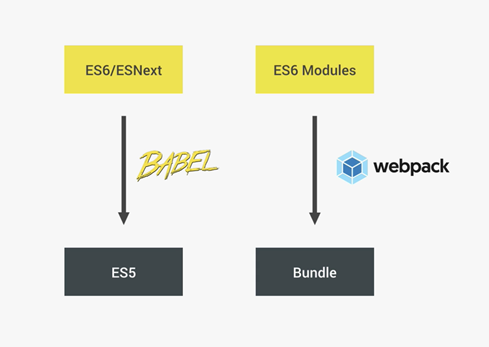
Babel – A Tool to convert ES6 to ES5
Babel is a toolchain that is mainly used to convert ECMAScript 2015+ code into a backwards-compatible version of JavaScript in current and older browsers or environments. Here are the main things Babel can do for you:
- Transform syntax
- Polyfill features that are missing in your target environment (through @babel/polyfill)
- Source code transformations (codemods)
Webpack – A Module Bundler
At its core, webpack is a static module bundler for modern JavaScript applications. When webpack processes your application, it internally builds a dependency graph which maps every module your project needs and generates one or more bundles.
The Core Concepts of Webpack are:
- Entry
- Output
- Loaders
- Plugins
- Mode
- Browser Compatibility
Conclusion
JavaScript, like most web-related technologies, is evolving all the time. Since the introduction of ES6, many awesome features have been added to it. Hope you liked the introduction bit and you begin to take advantage of these concepts to increase your JavaScript expertise. If you have any queries or concerns, please use the comment section below to let us know about them. Another blog in this series will be coming soon. Please stay tuned for it.