With the availability of many new tools and libraries for software development, knowing the benefits of these tools helps in choosing the right one for your business. Here is all about Redux – one app — one store — one state.
Redux in a nutshell
- A popular JavaScript library for managing the state of your application.
- A single application data container managed by the constraints of immutability.
- Works in synergy with React core of CDD (component Driven Development).
Why Redux?
React helps us to divide up our app into multiple components, and these components can communicate individually with each other, where data transfer between components is possible. But that doesn’t clearly specify how to keep track of the data (aka State), or how to manage the state object. In other words, React hasn’t provided any methodologies to structure data for bigger applications and this is where Redux comes up, as an appropriate way to fix the state management problem. It’s a complimentary library to React, that provides a way to easily store the data (State) and the events (Actions). Redux isolates the state object from the components.

Fundamental Principals of Redux
Single source of truth: The only state of the whole application is stored in an object tree within a single store. A single state tree makes it easier to debug or inspect an application.
In other words,
one app — one store — one state.
State Immutability: The state is read-only, and it cannot be changed directly. Instead, the state should be updated through an action, i.e., an object describing what has happened. This ensures that neither the views nor the network callbacks will ever be able to write directly to the state.
Changes are made with pure function: Pure functions here are the reducers, where the state gets updated without mutating the previous state by actions.
Building Blocks
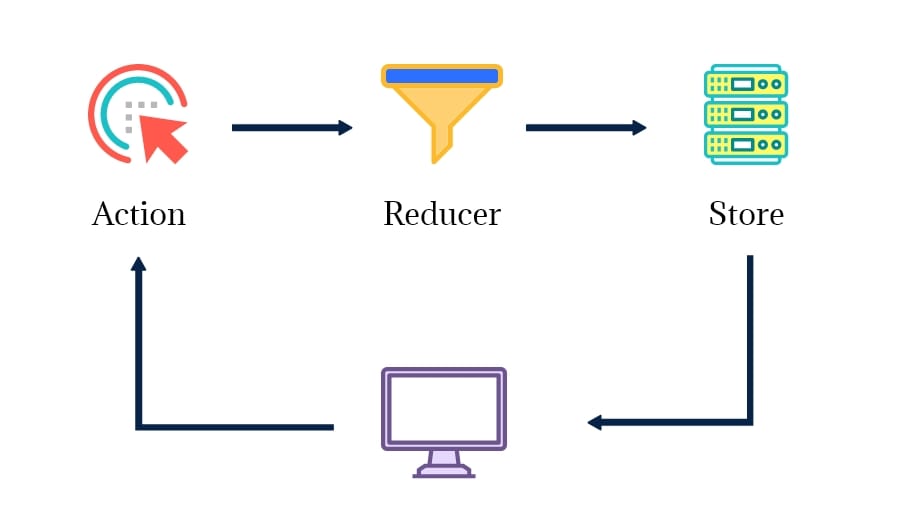
ACTION
Actions are plain JavaScript objects that describe what happens but don’t describe how the app state changes. Their job is to describe an event that took place in the application and to transport the new data to the stores. Each Action must have a type and an optional payload key that contains the data. An Action looks like the one below:
{ actionType: "UPDATE_TITLE", payload: "This is a new title." }
STORE
The Store is the object that brings Action and Reducer together. Store holds the state of the application.
createStore(rootReducer);
This createStore method is used for creating the application’s Store. It accepts three arguments: rootReducer, initialState and Redux middleware constant (the latter two being optional).
REDUCER
Reducers are pure functions that define how the app state changes. Whenever we dispatch an Action to our store, Redux passes down the Action to the Reducer. Then, the Reducer updates the state depending upon the Action that was passed. The Reducer function takes two arguments: the previous app state, the Action being dispatched and returns the new app state.
(previousState, action) => newState
REDUX Data Flow
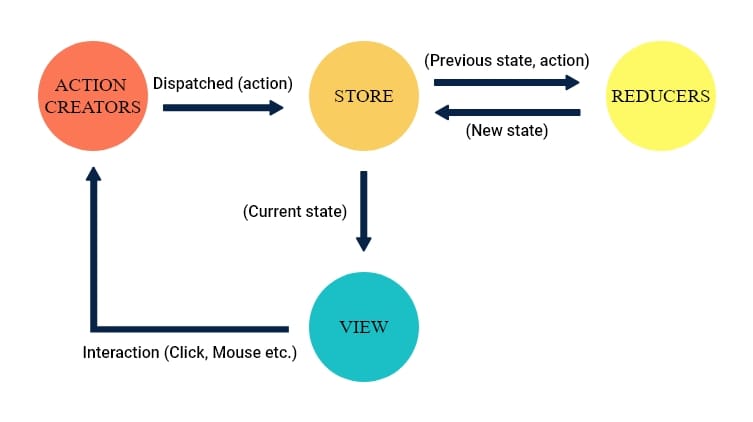
React and Redux don’t have a direct relationship—Redux controls an app’s state changes, while React renders the view of states.
When we integrate with React, we need React-Redux as a bridge library, which allows communication between Redux and React libraries. You can install the same by executing the following command in the command line,
npm i redux react-redux
To start off with connecting Redux and React, we will use Provider. It’s a React component provided by React-Redux. It makes our application store available throughout the entire app.
<Provider store={store}> <App/> </Provider>
CONNECT
Now that we have provided the Redux store to our application, we can connect our Redux Store to React components using the connects method. We just need to fire our action creators; all later logics are handled by the connect function.
You will need to use connect with two or three arguments, depending on the use case. The fundamental things to know are:
- mapStateToProps – This is the first argument and it helps with subscribing to the store by mapping store values in our component properties.
- mapDispatchToProps – This second argument has to do with injecting action creators in our component properties. But if you don’t want to inject any action, you can provide null as the second argument.
Basically, mapStateToProps and mapDispatchToProps are bothpure functions, that provide the store’s state and dispatch respectively. Furthermore, both functions must return an object, whose keys will then be passed on as the props of the component they are connected to.
Conclusion
In this article, we have discussed the fundamentals of Redux. Redux falls under the category of those libraries which seem to get more traction these days. Now that you know the basic building blocks, and how the state of an application is maintained, stay tuned for more on Redux.